layout に comment_list_row.xml を作成します。
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical" >
<LinearLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:orientation="horizontal" >
<TextView
android:id="@+id/commentNo"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="100"
android:textAppearance="?android:attr/textAppearanceMedium"
android:textColor="#55EEFF"
android:textSize="12dp"
android:textStyle="bold" />
<TextView
android:id="@+id/commentUserID"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="30dp"
android:text="12345678"
android:textAppearance="?android:attr/textAppearanceMedium"
android:textSize="12dp"
android:textStyle="bold" />
</LinearLayout>
<TextView
android:id="@+id/commentText"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="こんばんは"
android:textAppearance="?android:attr/textAppearanceMedium"
android:textSize="15dp" />
</LinearLayout>
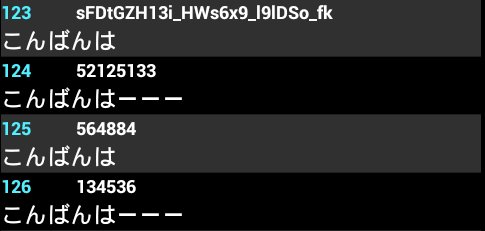
カスタム ListViewを作成します。BaseAdapterを拡張しました。
LayoutInflater を使用すると、 R.layout.comment_list_row が読み込めます。
import java.util.ArrayList;
import java.util.List;
import android.content.Context;
import android.graphics.Color;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.ListView;
import android.widget.TextView;
public class NicoCommentListView {
private ListView listView;
private CommentAdapter adapter;
public NicoCommentListView(ListView listView, Context context){
this.listView = listView;
adapter = new CommentAdapter(context, R.layout.comment_list_row);
setNicoCommentListView();
}
private void setNicoCommentListView(){
listView.setAdapter(adapter);
listView.setFastScrollEnabled(true);
}
public void append(String[] comment){
adapter.add(new CommentData(comment[0], comment[1], comment[2]));
adapter.notifyDataSetChanged();
listView.smoothScrollToPosition(listView.getCount());
}
private class CommentData {
public String No;
public String UserID;
public String Comment;
public CommentData(String no, String userID, String comment){
No = no;
UserID = userID;
Comment = comment;
}
}
private class CommentAdapter extends BaseAdapter {
private final int oddColor = Color.rgb(0, 0, 0);
private final int evenColor = Color.rgb(50, 50, 50);
private Context context;
private int textViewResourceId;
private List<CommentData> list = new ArrayList<CommentData>();
public CommentAdapter(Context context, int textViewResourceId) {
this.context = context;
this.textViewResourceId = textViewResourceId;
}
public void add(CommentData data){
list.add(data);
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
LayoutInflater inflater = (LayoutInflater)context.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
View view = inflater.inflate(textViewResourceId, null);
TextView tvNo = (TextView)view.findViewById(R.id.commentNo);
TextView tvUserID= (TextView)view.findViewById(R.id.commentUserID);
TextView tvComment= (TextView)view.findViewById(R.id.commentText);
tvNo.setText(list.get(position).No);
tvUserID.setText(list.get(position).UserID);
tvComment.setText(list.get(position).Comment);
if (position%2 == 0) {
view.setBackgroundColor(evenColor);
}
else {
view.setBackgroundColor(oddColor);
}
return view;
}
@Override
public int getCount() {
return list.size();
}
@Override
public Object getItem(int position) {
return list.get(position);
}
@Override
public long getItemId(int position) {
return list.indexOf(list.get(position));
}
}
}
Configuring VM Acceleration on Linux
- Android SDK Manager
Android 4.0.3 (API15)と Android 2.3.3 (API10)には、Intel x86 Atom System Image があるので、追加インストールします。
Windows の場合は、Intel Hardware Accelerated Execution Manager も必要です。
- Android Virtual Device Manager (Tools Manage AVDs...)
- Name:Android403API15x86
- Target:Android 4.0.3 - API Level 15
- CPU/ABI:Intel Atom (x86)
- 起動用のScriptを作成してEmulatorを起動します。
$ gedit avd403x86
----------------------------------------------------------------------------------
#!/bin/sh
android-sdk-linux/tools/emulator -avd Android403API15x86 -qemu -m 512 -enable-kvm
----------------------------------------------------------------------------------
$ chmod a+x avd403x86
$ ./avd403x86
Windowsはこちらを参照
source github
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
btnLoginAlert = (Button)findViewById(R.id.btn_loginAlert);
btnLoginAlert.setOnClickListener(new LoginAlert());
etResponse = (EditText)findViewById(R.id.ed_response);
nicoMesssage = new NicoMessage();
nico = new NicoRequest(nicoMesssage);
}
class LoginAlert implements OnClickListener, Handler.Callback ,OnReceiveListener {
@Override
public void onClick(View v) {
key();
//ログインボタンをdisableにする
btnLogin.setEnabled(false);
btnLoginAlert.setEnabled(false);
final Handler handler = new Handler(this);
nicosocket = new NicoSocket(nicoMesssage);
nicosocket.setOnReceiveListener(this);
new Thread (new Runnable(){
public void run() {
nico.loginAlert(email.getText().toString(),password.getText().toString());
nicosocket.connectCommentServer(nico.getAlertAddress(), nico.getAlertPort(), nico.getAlertThread());
Message message = handler.obtainMessage(R.id.btn_loginAlert);
handler.sendMessage(message);
}}).start();
}
@Override
public boolean handleMessage(Message msg) {
if(nicosocket.isConnected()){
new Thread(nicosocket.getAlertSocketRun()).start();
btnLogin.setVisibility(View.GONE);
btnLoginAlert.setVisibility(View.GONE);
}else{
Toast.makeText(getApplicationContext(), "アラートログインに失敗しました", Toast.LENGTH_SHORT).show();
//ログインボタンをenableにする
btnLogin.setEnabled(true);
btnLoginAlert.setEnabled(true);
}
return true;
}
@Override
public void onReceive(String receivedMessege) {
etResponse.append(receivedMessege + "\n");
}
}
Android 2.3では動作していたのに, Android 4(ICS)では動作しなくなったときの対応です。
3.0 からView設計が変更されたらしい。
1.1 NetworkOnMainThreadException メインのスレッドから時間がかかる処理を外せってことみたいです。 なので別のスレッドを走らせるようにします。 GUIが反応しないのを防ぐようにとAndroidのView設計が変わったみたいです。 時間がかかる処理の例 POSTやGET SQLよるデータ処理 Fileの読み込みや書き込み 1.2 CalledFromWrongThreadException 別スレッドでVIewにあるテキストなどの変更をするなということみたい。 HandlerとMessageを使って処理終了のやり取りをする。 まあViewにイベント処理として扱えばいい。
githubにコードを載せました。
EclipseとAndroid Emulatorを別々のPCで起動してデバックできないかを試してみました。
エミュレータとの接続に使われるLocal portを別のPCにforwardさせるスクリプトファイルを作成します。
sshサーバとエミュレータが動いているのPCは同一でIPアドレスが、192.168.0.xの場合
chadb
------------------------------
#!/bin/sh
ssh -N -f -L 5554:localhost:5554\
-L 5555:localhost:5555\
userName@192.168.0.x
------------------------------
スプリクトを読み込みます。
$ . chabd
エミュレータを起動させておきます。
うまくローカルポートが、エミュレータが動いているPCのローカルポートに、フォーワード(転送)されているかを確認します。
$ android-sdk-linux/platform-tools/adb devices
List of devices attached
emulator-5554 device
Eclipseのデバック設定をManualにします。
下記のように別のPCのエミュレータが表示されているので選択してデバックします。
$ mkdir ICS-x86
$ cd ICS-x86
$ repo init -u https://android.googlesource.com/platform/manifest -b android-4.0.3_r1
$ repo sync
$ . build/envsetup.sh
$ lunch full_x86_eng
$ make -j8
Processing target/product/generic/obj/APPS/Settings_intermediates/package.apk
Done!
Install: out/target/product/generic/system/app/Settings.odex
Install: out/target/product/generic/system/app/Settings.apk
Finding NOTICE files: out/target/product/generic/obj/NOTICE_FILES/hash-timestamp
Combining NOTICE files: out/target/product/generic/obj/NOTICE.html
Installed file list: out/target/product/generic/installed-files.txt
Target system fs image: out/target/product/generic/obj/PACKAGING/systemimage_intermediates/system.img
Install system fs image: out/target/product/generic/system.img
Android x86 をビルドしました。
不足しているライブラリを下記します。
- /usr/include/gnu/stubs.h:7:27: error: gnu/stubs-32.h: No such file or directory
libc6-dev-i386" - -lstdc++:g++-multilib libc6-dev-i386
- -lz:lib32z-dev
- -lncurses:lib32ncurses5-dev lib32readline5-dev
不足ライブラリをインストールします。
sudo apt-get install libstdc++6-4.5-dev g++-multilib libc6-dev-i386 lib32ncurses5-dev lib32readline5-dev
Fedora14 x86_64 でビルドしたため x86 のライブラリをインストールしました。
クロスコンパイルですね。下記から検索します。
http://rpm.pbone.net/index.php3
- /usr/bin/ld: cannot find -lXi
libXi
------------------------------------------------------
$ make xconfig
HOSTLD scripts/kconfig/qconf
/usr/bin/ld: cannot find -lXi
collect2: ld はステータス 1 で終了しました
make[1]: *** [scripts/kconfig/qconf] エラー 1
make: *** [xconfig] エラー 2
------------------------------------------------------- - /usr/bin/ld: cannot find -lz
zlib-devel - /usr/bin/ld: skipping incompatible /usr/lib64/libtinfo.so when searching for -ltinfo
/usr/bin/ld: cannot find -ltinfo
ncurses-devel - libreadline.so
readline-devel